Intro
Fake cloud shadows are a great way to add depth to your scene, providing the illusion of high-quality environmental shading for negligible cost and effort. Below is an example from Warhammer 3, where it's apparent in the sped-up footage the clouds in the sky are looping/repeating and don't match the actual shadows on the ground.
Core
There are a few ways to accomplish this in Unity, but one highly versatile solution is to use an animated/scrolling texture as a light cookie on any light source. Thus, your clouds are directly masking your "sun". This simple technique has many uses.
You need only two things for the most simple implementation:
A tiling 'cloud' (noise) texture.
A custom component to animate the cookie offset (scrolling the UVs).
Implementation
Here’s the code for LightCookieScrollUV.cs
.
using UnityEngine;
using UnityEngine.Rendering.Universal;
[ExecuteAlways] // Run in the editor.
public class LightCookieScrollUV : MonoBehaviour
{
// In URP, we need to scroll the light cookie.
// -> lightCookieOffset in URP's UniversalAdditionalLightData component.
UniversalAdditionalLightData lightData;
public Vector2 speed;
void Start()
{
}
void Update()
{
// If null, assign lightData.
if (!lightData)
{
lightData = GetComponent<UniversalAdditionalLightData>();
}
// Scroll UVs of the light cookie texture.
lightData.lightCookieOffset = speed * Time.time;
}
}
Make sure to attach it directly to your light source. That’s it!
Future
You can also take advantage of animating light cookies for something like caustics.
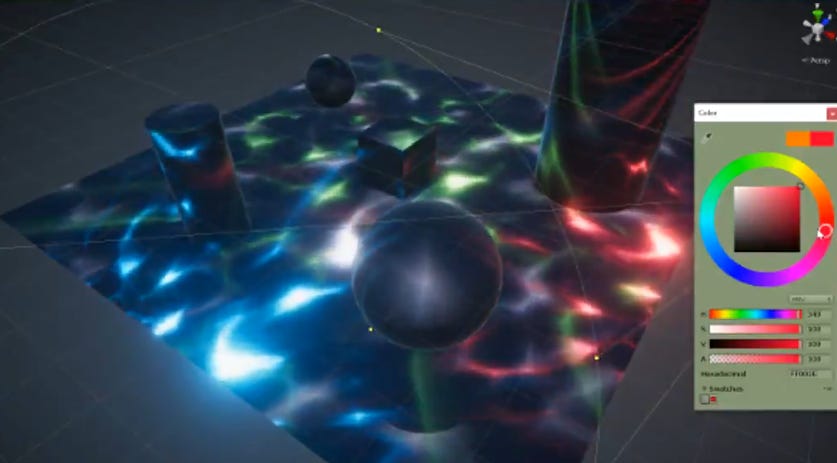
It’s possible to synchronize the light’s projection with actual volumetric rays using raymarching off the same texture. Here’s an example from my earlier experiments.
An alternative method is using a post-processing effect which uses the surface position of the scene’s geometry to sample a texture or noise function, like I did here.
Perhaps in the future I can cover these more advanced implementations.
You can follow me on Twitter/X for more (@TheMirzaBeig)!